오랜만입니다..ㅎㅎ
DOTween
C#에 최적화된 객체 지향적 애니메이션 엔진으로 다양한 애니메이션을 간단한 코드 한 줄로 손쉽게 구현할 수 있다.
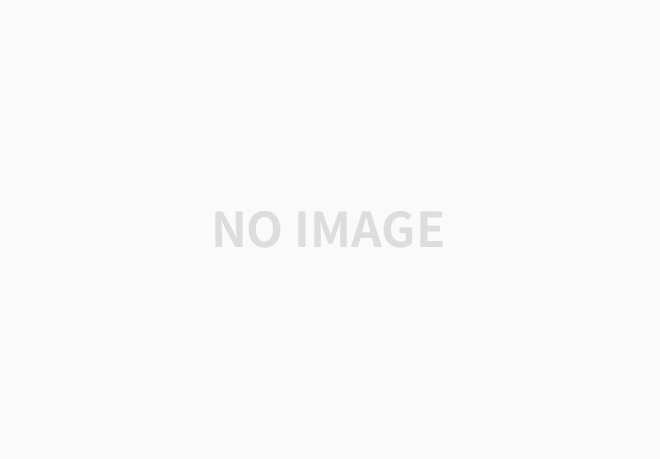
무료 버젼
DOTween (HOTween v2) | 애니메이션 도구 | Unity Asset Store
Use the DOTween (HOTween v2) tool from Demigiant on your next project. Find this & more animation tools on the Unity Asset Store.
assetstore.unity.com
유료 버젼
Visual Path Editor, 스크립트 없이 애니메이션 적용 가능등 프로에만 지원되는 기능들이 있다.
DOTween Pro | 비주얼 스크립팅 | Unity Asset Store
Get the DOTween Pro package from Demigiant and speed up your game development process. Find this & other 비주얼 스크립팅 options on the Unity Asset Store.
assetstore.unity.com
에셋을 import하고 꼭 Tools > DOTween Utility Panel 실행 후 Setup DOTween 클릭해야 한다!
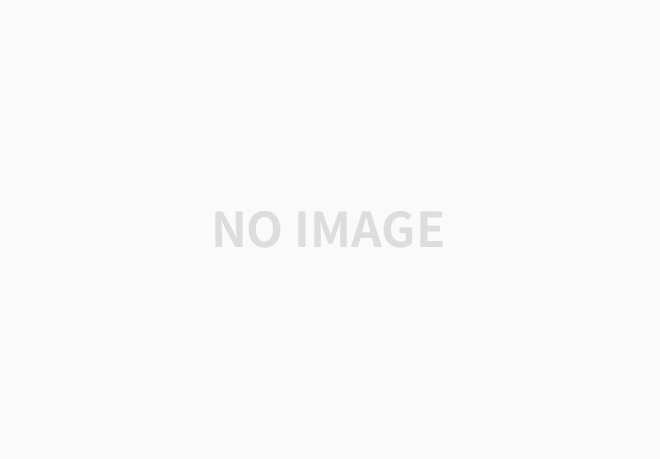
먼저 DOTween을 사용하려면 using문을 추가해야한다.
using DG.Tweening;
이제 코드 비교를 통해 DOTween이 얼마나 편한지 알아보자
1. 위치 이동
보통 자연스러운 위치 이동을 위해 Lerp을 사용하였다.
01 Lerp을 사용하는 경우
using UnityEngine;
public class lerpExample : MonoBehaviour
{
public Vector3 targetPosition = new Vector3(5, 0, 0);
private Vector3 startPosition;
private float time = 0f;
private float duration = 2f;
void Start()
{
startPosition = transform.position;
}
void Update()
{
if (time < duration)
{
time += Time.deltaTime;
float t = time / duration; // 0에서 1 사이 값
transform.position = Vector3.Lerp(startPosition, targetPosition, t);
}
}
}
02 DOTween을 사용하는 경우
using UnityEngine;
using DG.Tweening;
public class dOTweenExample : MonoBehaviour
{
void Start()
{
transform.DOMove(new Vector3(5, 0, 0), 2f);
}
}
DOMove 매개변수
ublic static TweenerCore<Vector3, Vector3, VectorOptions> DOMove(this Transform target, Vector3 endValue, float duration, bool snapping = false);
target : 대상 객체
endValue : 목표 위치
duration : 이동하는데 걸리는 시간
snapping : 보간 여부(기본은 true로 설정되어있음)
2. 회전
using DG.Tweening;
void Start()
{
transform.DORotate(new Vector3(0, 180, 0), 2f);
}
DORotate 매개변수 설명
public static TweenerCore<Quaternion, Vector3, QuaternionOptions> DORotate(this Transform target, Vector3 endValue, float duration, RotateMode mode = RotateMode.Fast);
target : 대상 객체
endValue : 목표 회전 값
duration : 걸리는 시간
mode : 회전모드 설
3. 크기 변경
using DG.Tweening;
void Start()
{
// 2초 동안 크기를 2배로 증가
transform.DOScale(new Vector3(2f, 2f, 2f), 2f);
}
DOScale 매개변수
public static TweenerCore<Vector3, Vector3, VectorOptions> DOScale(this Transform target, Vector3 endValue, float duration);
target : 대상 객체
endValue : 최종 크기
duration : 진행할 시간
4. 색상 변경
using DG.Tweening;
void Start()
{
// 2초 동안 색상을 빨간색으로 변경
GetComponent<Renderer>().material.DOColor(Color.red, 2f);
}
DOColor 매개변수
public static TweenerCore<Color, Color, ColorOptions> DOColor(this Material target, Color endValue, float duration);
target : 대상 객체
endValue : 목표 색상
duration : 몇초동안 색상을 바꿀 것인지
5. 타이핑 효과
보통 글자 타이핑효과를 넣을때 코루틴을 사용하는데
using UnityEngine;
using UnityEngine.UI;
using System.Collections;
public class example : MonoBehaviour
{
public Text text;
private string targetText = "Hello, World!";
private float duration = 3f;
void Start()
{
StartCoroutine(AnimateText());
}
IEnumerator AnimateText()
{
string text = "";
float time = 0f;
while (time < duration)
{
timer += Time.deltaTime;
int count = Mathf.FloorToInt((time / duration) * targetText.Length);
currentText = targetText.Substring(0, count);
myText.text = text;
yield return null;
}
myText.text = targetText;
}
}
만약 DOTween을 사용한다면 아래와 같이 한줄로 적을 수 있다.
using DG.Tweening;
using UnityEngine;
using UnityEngine.UI;
public class DOTweenTextAnimation : MonoBehaviour
{
public Text myText; // Unity UI Text 컴포넌트
void Start()
{
myText.DOText("Hello, World!", 3f);
}
}
DOText 매개변수
public static TweenerCore<string, string, StringOptions> DOText(this Text target, string endValue, float duration, bool richTextEnabled = false);
target : 변경할 텍스트
endValue : 애니메이션이 끝날때 최종 텍스트
duration : 걸리는 시간
richTextEnabled : bold체나 기울임을 설정할 수 있다.
6. 트윈 옵션
제일로 좋은 장점 중 하나가 트윈 옵션이다!
01 SetEase
애니메이션의 속도 변화 방식을 설정
transform.DOScale(new Vector3(2f, 2f, 2f), 2f).SetEase(Ease.InOutQuad);
02 SetLoops()
애니메이션의 반복 설정
transform.DOScale(new Vector3(2f, 2f, 2f), 2f).SetLoops(-1, LoopType.Yoyo);
03 SetDelay()
딜레이 추가
transform.DOScale(new Vector3(2f, 2f, 2f), 2f).SetDelay(1f);
04 OnComplete()
애니메이션 완료 후 실행할 코드 추가
transform.DOScale(new Vector3(2f, 2f, 2f), 2f).OnComplete(() => Debug.Log("애니메이션 완료!"));
05 다양하게 섞어서도 사용가능
transform.DOMoveX(5, 2f)
.SetEase(Ease.InOutQuad) // 부드러운 가속/감속 효과
.SetLoops(2, LoopType.Yoyo) // 왕복 애니메이션 (2번 반복)
.SetDelay(1f) // 1초 지연 후 실행
.OnComplete(() => Debug.Log("애니메이션 완료!")); // 완료 후 실행
'유니티 공부 > Unity' 카테고리의 다른 글
Unity - 쉐이더 분홍색(핑크색) 오류 해결 방법 (1) | 2025.03.13 |
---|---|
Unity - 에셋 추천 Trails FX(잔상 효과, 검 효과, 발자국 등) (0) | 2025.03.12 |
Unity - DirtyFlag Pattern (0) | 2024.11.11 |
Unity - Flyweight Pattern(+) ScriptableObject) (0) | 2024.11.09 |
Unity - Sprite Library Asset (0) | 2024.11.08 |
댓글